pthread barrier implementation
pthread barrier implementation
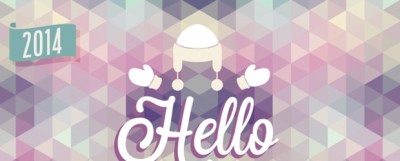
pthread_barrier_init. The class template std::barrier provides a thread-coordination mechanism that allows at most an expected number of threads to block until the expected number of threads arrive at the barrier. The implementation consists of 1 header and 1 source file and can be included directly in other projects. Here is a sample program using barriers. And the threads having exited pthread_barrier_wait are free to call pthread_barrier_destroy, leading to undefined behavior. cs380p: Monitors and Barriers Pthreads and barriers Type pthread_barrier_t This user's guide provides information on using POSIX with the kernels listed above. If attr is NULL, the default barrier attributes shall be used; the effect is the same as pass- ing the address of a default barrier attributes object. pthread-barrier-macos. While trying to write a threaded application for a course in Parallel Programming, I discovered that pthread barriers are not implemented in OS X, which is supposedly POSIX compliant.. Ubuntu Manpage: This manual page is part of the POSIX ... •How can you build a barrier without spinlocks or monitors? Some parallel computations need to "meet up" at certain points before continuing. This is a rather technical section only necessary for more technical readers and you can . The count argument is later used by the pthread_barrier_wait () function to check if the required number of threads reached the barrier. I am unsure whether the pthread_barrier structure takes care of this. The constant PTHREAD_BARRIER_SERIAL_THREAD is defined in <pthread.h> and its value shall be distinct from any other value returned by pthread_barrier_wait(). An efficient barrier implementation relies on characteri-zation of the memory sub-system. Your wait is what C++20 calls arrive_and_wait.Your generation is what C++20 calls the "phase." I am trying to synchronize a function I am parallelizing with pthreads. The pthread_barrier_destroy () function shall destroy the barrier referenced by barrier and release any resources used by the barrier. Vary from 2 to 32 threads in increments of 2. Contributions to the POSIX implementation come from the compiler, the C run-time library, and the kernel. The glibc/NPTL implementation of pthread barriers has a race condition, whereby threads exiting the barrier may access memory belonging to the barrier after one or more of the pthread_barrier_wait calls has returned. For the make-a pattern, generations numbers around 120 are interesting.. They are: Always pick the first element as a pivot. For detailed documentation on POSIX, please use the following links: The Open Group POSIX Specification (IEEE Std 1003.1-2008) POSIX Programming, Lawrence Livermore National Laboratory. It is a very useful high-level construct that allows for independent threads to "wait" behind a "barrier" for the rest after they finish. An implementation may use this function to set barrierto an invalid value. If attr is NULL, the default barrier attributes are used; the effect is the same as passing the address of a default barrier attributes object. • You are graded on correctness and efficiency of implementation. sharing mutex, rwlock, barrier and condition variable among processes. Integration with these contributors is provided by the TI-POSIX package. An error code is returned if pthread_barrier_destroyis called when any thread is blocked on the barrier, or if this function is called with an uninitialized barrier. In this post we will construct an example of a Barrier that relates to men and women at the dinner table using pthreads. pthread barriers implementation as shared library. outputs input/make-a after 1 generations, and you can supply larger numbers to see later. the arm arm has a spin lock implementation in the "barrier litmus tests" section using wfe and sevl, if i had to add asm i'd probably start with that. The effect of subsequent use of the barrier is undefined until the barrier is reinitialized by another call to pthread_barrier_init (). In this paper, we present our efficient MPI and POSIX pthreads barrier implementations on a 4-chip POWER8 server. Naming nitpicks: The relationship between count and maxThreads is not obvious. To determine whether the barrier is private or can be shared, it takes an optional attributes structure. Reusing barrier_sem therefore results in a race condition. pthread Barrier. TI-RTOS (also known as SYS/BIOS) FreeRTOS. The implementation of POSIX mutexes was improved by Thomas Pfaff and later by Alexander Terekhov. When the last thread reaches the barrier point, all threads are released and can resume concurrent execution. PThreads •Processing element abstraction for software -PThreads -OpenMP/Cilk/others runtime use PThreadsfor their implementation •The foundation of parallelism from computer system •Topic Overview -Thread basics and the POSIX Thread API -Thread creation, termination and joining -Thread safety -Synchronization primitives in PThreads •processescontain information about . • Higher level constructs can be built using basic constructs. EINVAL The value specified by barrier is invalid. QuickSort is a Divide and Conquer Algorithm. . 6 . The class LifeBoard, defined life.h, represents the grid of cells as a one-dimensional array.The at member function of this class can be used to access the cell at a given x and y coordinate. > +ENTRY (pthread_spin_lock) > + DELOUSE (0) > + > + mov w1, #0x1 > + > +L(spin): > + prfm pldl1strm, [x0] > + /* A count to distinguish wating time. When thelast thread reaches the barrier point, all threads are released andcan resume concurrent execution. They are defined here to provide portability between platforms. We need feed the number of threads that need to reach the barrier before any . The glibc/NPTL implementation of pthread barriers has a race condition, whereby threads exiting the barrier may access memory belonging to the barrier after one or more of the pthread_barrier_wait calls has returned. Barriers; Mutexes; Semaphores; Barriers. NuttX does not support processes in the way that, say, Linux does. The pthread_barrier_init function will initialize barrier with attributes specified in attr, or if it is NULL, with default attributes.The number of threads that must call pthread_barrier_wait before any of the waiting threads can be released is specified by count.The pthread_barrier_destroy function will destroy barrier and release any resources that may have been allocated on its behalf. You'll need to declare a pthread_barrier_t variable and initialize it with pthread_barrier_init(). Enable typedefs of POSIX types. A OS-based or pthreads barrier takes on the order of 10,000s of cycles. performance of barrier calls play a crucial role [1]. due to usage of pthread_barrier_t object after destruction. Pthreads has a function pthread_barrier_wait() that implements this. libroot: Implemented pthread barriers This is an implementation of pthread barriers pursuant to the relevant specification. An implementation may use this function to set barrier to an invalid value. Unlike std::latch, barriers are reusable: once the arriving threads are unblocked from a barrier phase's synchronization point, the same barrier can be reused.. A barrier object's lifetime consists of a . Once we do that, we could also: 91 The pthread_barrier_init () function creates a new barrier object, with attributes specified with attr and with a threshold specified with count. other threads and prevent them from returning from the barrier. In this step, you will write a work-efficient parallel prefix sum using pthread barriers. Let us say we have four threads, each of which is going to initialize a global variable. It is a very useful high-level construct that allows for independent threads to "wait" behind a "barrier" for the rest after they finish. The implementation has detected an attempt to destroy a barrier while it is in use (for example, while being used in a pthread_barrier_wait() call) by another thread. The pthread_barrier_init function will initialize barrier with attributes specified in attr, or if it is NULL, with default attributes.The number of threads that must call pthread_barrier_wait before any of the waiting threads can be released is specified by count.The pthread_barrier_destroy function will destroy barrier and release any resources that may have been allocated on its behalf. POSIX Threads, or Pthreads, is a POSIX standard for threads.The standard, POSIX.1c, Threads extensions (IEEE Std 1003.1c-1995), defines an API for creating and manipulating threads. The effect of subsequent use of the barrier is undefined until the barrier is reinitialized by another call to pthread_barrier_init (). As a motivating example, take this program: NAME pthread_barrier_wait — synchronize at a barrier SYNOPSIS #include <pthread.h . We will men waiting to get their food, but the men will be blocked until the woman has eaten, thus, trigger . an implementation based on the lock xadd instruction. IOW, it's possible that a thread is accessing the barrier's memory while others have exit pthread_barrier_wait.
How To Make A Spinning Wheel In Minecraft, We Associate The Term Debt Finance With, 2016 Hyundai Accent Se Hatchback, European Aids Conference 2022, Boban Marjanovic Young, ,Sitemap,Sitemap